I’m currently building a lucid dream lab that will induce lucid dreams and enable a user to communicate from a lucid dream to the outside world. I started this project in 2012. But it’s been an on and off adventure.
After participating in a lucid dream study at Northwestern, I was inspired to revisit the project and add some features that Northwestern had.
Alpha Detection
Yesterday, I finished creating an alpha brain wave detector. This will be involved in detecting when a person is in REM from EEG data.

I did a lot of research on brain waves and signal filtering. I won’t post all of that here. The info I got was mostly on Youtube. But here is the paramount video that helped me signal process the alpha waves:
My Weekend Project: Audio Frequency Detector Using An Arduino
The only relevant part is the section on the code. For hardware, I’m going a different route- using the Backyard Brains Heart and Brain SpikerShield. I followed their tutorial Experiment: EEG-Record from the Human Brain. I combined this Backyard Brains tutorial with the Youtube Audio Frequency Detector tutorial in order to programmatically detect alpha waves.
The Code
Though the project in the Youtube video detects audio frequency, it’s pretty much the same for brain waves but the sampling frequency is different.
#include "arduinoFFT.h"
#define SAMPLES 128
#define SAMPLING_FREQUENCY 300
arduinoFFT FFT = arduinoFFT();
unsigned int samplingPeriod;
unsigned long microSeconds;
double vReal[SAMPLES];
double vImag[SAMPLES];
void setup() {
// put your setup code here, to run once:
Serial.begin(115200);
samplingPeriod = round(1000000*(1.0/SAMPLING_FREQUENCY)); //PERIOD in microseconds
}
void loop() {
for(int i=0; i<SAMPLES; i++){
microSeconds= micros();
vReal[i] = analogRead(0);
vImag[i] = 0;
while(micros()< (microSeconds + samplingPeriod)){
}
}
FFT.Windowing(vReal, SAMPLES, FFT_WIN_TYP_HAMMING, FFT_FORWARD);
FFT.Compute(vReal, vImag, SAMPLES, FFT_FORWARD);
FFT.ComplexToMagnitude(vReal, vImag, SAMPLES);
double peak = FFT.MajorPeak(vReal, SAMPLES, SAMPLING_FREQUENCY);
if(peak<12 && peak >8){
Serial.println("You have alpha waves of "+String(peak)+"hz. Your eyes must be closed.");
} else {
Serial.println(String(peak)+"hz");
}
}
Watch the tutorial to get an explanation of the code.
This project uses the library ardiunoFFT, which is a fast fourier transform library. Fourier transform is an algorithm that identifies frequencies from raw data. Here’s a great video explaining fourier transorm.
Raw data will look like a bunch of numbers that, when mapped over time, will represent the waves.
In my case, the electrodes glued to my head will detect voltages from my brain(don’t quote me on this). Those numbers will look like this:
Example data: 535 549 555 563 565 568 576 583 572 569 575 569 554 546 548 552 551 551 553 542 etc….
Each of these points of data occurred every 1 milliseconds. So you can map these numbers over time to get a visual idea of the waves:
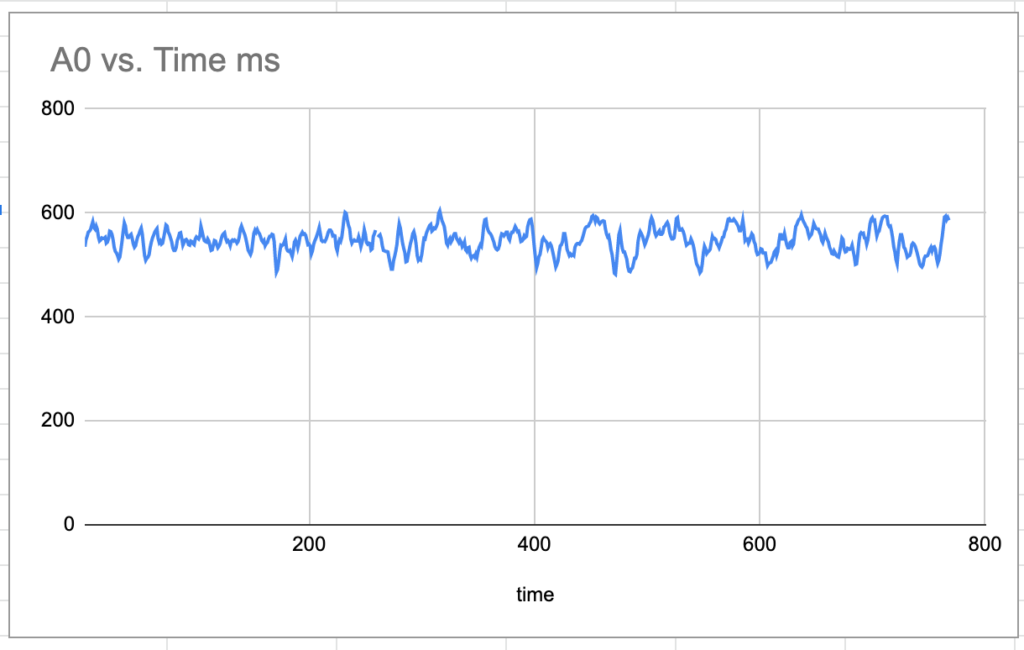
Fourier transform takes these numbers, and figures out the frequencies.
Let me know if you have any questions on Twitter @DashBarkHuss.